If you’ve encountered the frustrating error message “ModuleNotFoundError: No module named ‘RVTools'”, you’re not alone. Whether you’re a seasoned Python developer or someone working in the world of IT automation, such an error can bring your scripts to a screeching halt. But what exactly causes this error, and more importantly, how can you resolve it quickly and effectively?
This article is your complete guide to understanding and resolving this error while shedding light on what RVTools is and how it interacts with Python.
Table of Contents
Understanding the ‘ModuleNotFoundError’
In Python, a ModuleNotFoundError occurs when the Python interpreter cannot locate a module you’re trying to import. This usually happens when:
- The module is not installed in your environment
- There is a typo in the module name
- The package does not exist in a pip-installable form
In the case of ‘RVTools’, the issue is particularly unique because RVTools is primarily a Windows-based GUI application developed by Rob de Veij for retrieving information from VMware environments. It is not a standard Python module available on PyPI. This causes confusion when developers attempt to import it directly in Python.
Clarifying the Confusion: RVTools vs. Python Module
It’s important to recognize that RVTools is not a Python library. It is a Windows executable that exports data (such as VM details, datastores, and snapshots) mostly in Excel or CSV format. Attempting to install it via pip will naturally result in an error like:
pip install RVTools
ERROR: Could not find a version that satisfies the requirement RVTools
So if you’re getting ModuleNotFoundError when trying to use RVTools with Python, it’s likely because you’re using a script that expects RVTools data (like an exported CSV file) or that integrates with it through other methods, such as PowerShell or COM automation.
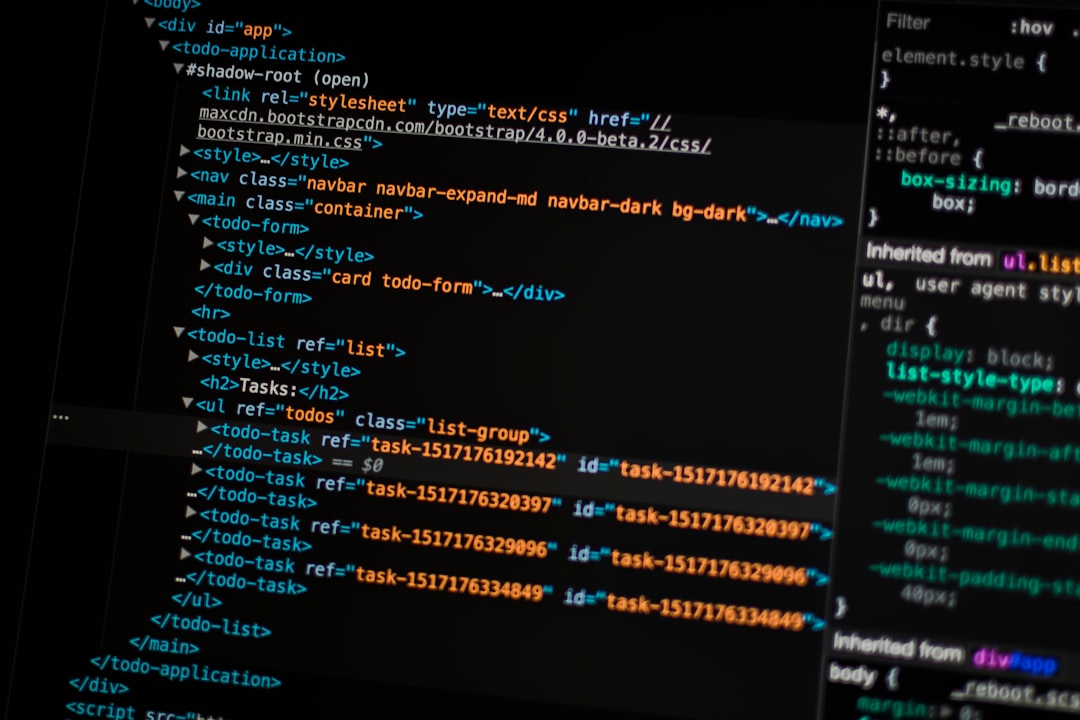
Practical Solutions to the Problem
To fix this issue, you can follow several approaches based on your actual use case:
1. Use RVTools Externally and Import the Data
The most straightforward method is to run RVTools manually or via a PowerShell script and export the data into CSV format. Then, import that CSV into Python.
import pandas as pd
df = pd.read_csv("RVToolsExport.csv")
print(df.head())
This method doesn’t require the RVTools module in Python. It only assumes that you have the exported data you need.
2. Automate RVTools Export with PowerShell
RVTools offers CLI options such as:
RVTools.exe -c ExportAll2csv -d ExportPath
You can call this in Python using the subprocess
module:
import subprocess
subprocess.run(["RVTools.exe", "-c", "ExportAll2csv", "-d", "C:\\Exports"])
This bridges the gap between Python and RVTools without requiring a direct Python module.
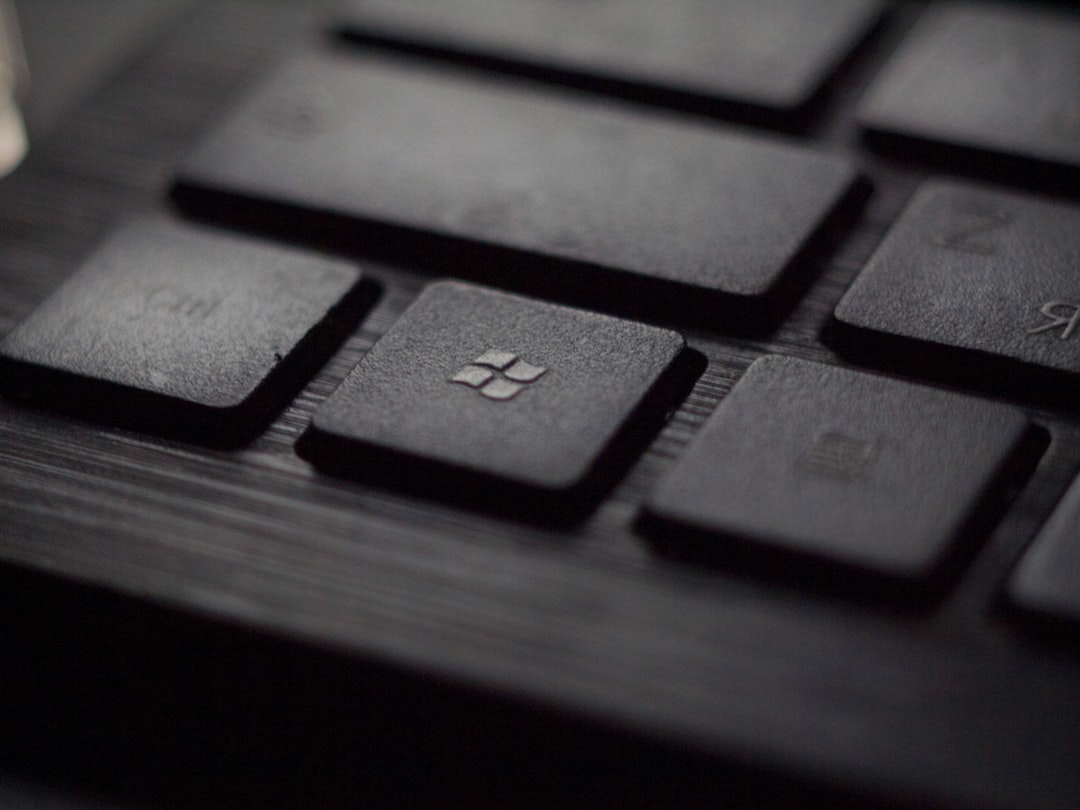
3. Use VMware APIs and SDKs Instead
If you’re scripting extensively in Python and want to avoid juggling GUI tools and exports, consider using the pyVmomi library. Developed by VMware, this library gives direct programmatic access to vCenter servers.
pip install pyvmomi
With this, you can query vSphere environments in real-time using Python, replacing the need for RVTools entirely for most use cases.
Common Mistakes and How to Avoid Them
A few common errors developers make when working with RVTools and Python include:
- Assuming RVTools has a Python API
- Using RVTools in environments where GUI apps can’t run
- Not automating the export process and manually importing data
Clarifying your goals—whether it’s automation, data extraction, or visualization—can help you choose the right tool or integration method.
Conclusion
While RVTools is a powerful utility for VMware data extraction, it’s not a Python module and cannot be imported in your scripts directly. The ModuleNotFoundError: No module named ‘RVTools’ error is a sign that you need an alternate approach—either by importing exported data, automating via PowerShell, or switching to native Python SDKs for VMware like pyVmomi.
Hopefully, this guide has helped clear up the confusion and pointed you toward a solution that aligns with your technical needs and project scope.