When working with WordPress loops and custom queries, it’s essential to ensure that global WordPress variables remain in their expected state. One of the key functions that help maintain order in your code is wp_reset_postdata()
. Understanding when and why to use this function can prevent potential issues in rendering posts and improve the reliability of your WordPress site.
Table of Contents
Understanding WP_Query
and Its Impact
WordPress provides the WP_Query
class to fetch custom post data. However, since it manipulates the global $post
variable, failure to reset post data can lead to unintended behavior. If you use multiple loops on a page, forgetting to call wp_reset_postdata()
may cause conflicts between queries, affecting post content and template rendering.
When Should You Use wp_reset_postdata()
?
There are specific scenarios where using this function is necessary to ensure smooth execution of your WordPress loops. Below are the most common cases where wp_reset_postdata()
should be used:
1. After a Custom WP_Query
Whenever you create a new instance of WP_Query
, you should reset post data after completing the loop. This prevents the custom query from affecting subsequent post content.
$args = array(
'post_type' => 'post',
'posts_per_page' => 5
);
$custom_query = new WP_Query($args);
if ($custom_query->have_posts()) {
while ($custom_query->have_posts()) {
$custom_query->the_post();
echo '' . get_the_title() . '
';
}
}
wp_reset_postdata(); // Reset global $post
Without calling wp_reset_postdata()
, the global $post
variable remains stuck at the last post in your custom query, potentially altering content displayed after the loop.
2. When Using Multiple Queries on the Same Page
WordPress pages often require multiple loops to display different types of content such as latest articles, featured posts, or custom post types. Without resetting post data, the output of subsequent sections might inherit unexpected values from a previous query.
$featured_posts = new WP_Query(array('category_name' => 'featured', 'posts_per_page' => 3));
if ($featured_posts->have_posts()) {
while ($featured_posts->have_posts()) {
$featured_posts->the_post();
echo '' . get_the_title() . '
';
}
}
wp_reset_postdata();
$latest_posts = new WP_Query(array('posts_per_page' => 5));
if ($latest_posts->have_posts()) {
while ($latest_posts->have_posts()) {
$latest_posts->the_post();
echo '' . get_the_title() . '
';
}
}
wp_reset_postdata();
Resetting post data after each query ensures that every loop starts fresh, preventing unintended carryovers between queries.
3. When Using Custom Queries Inside Templates
Theme and plugin developers often include custom queries inside template files to display specific content sections. If the reset function is omitted, it can interfere with default post loops defined in the main theme structure, leading to inconsistencies in how posts are displayed.
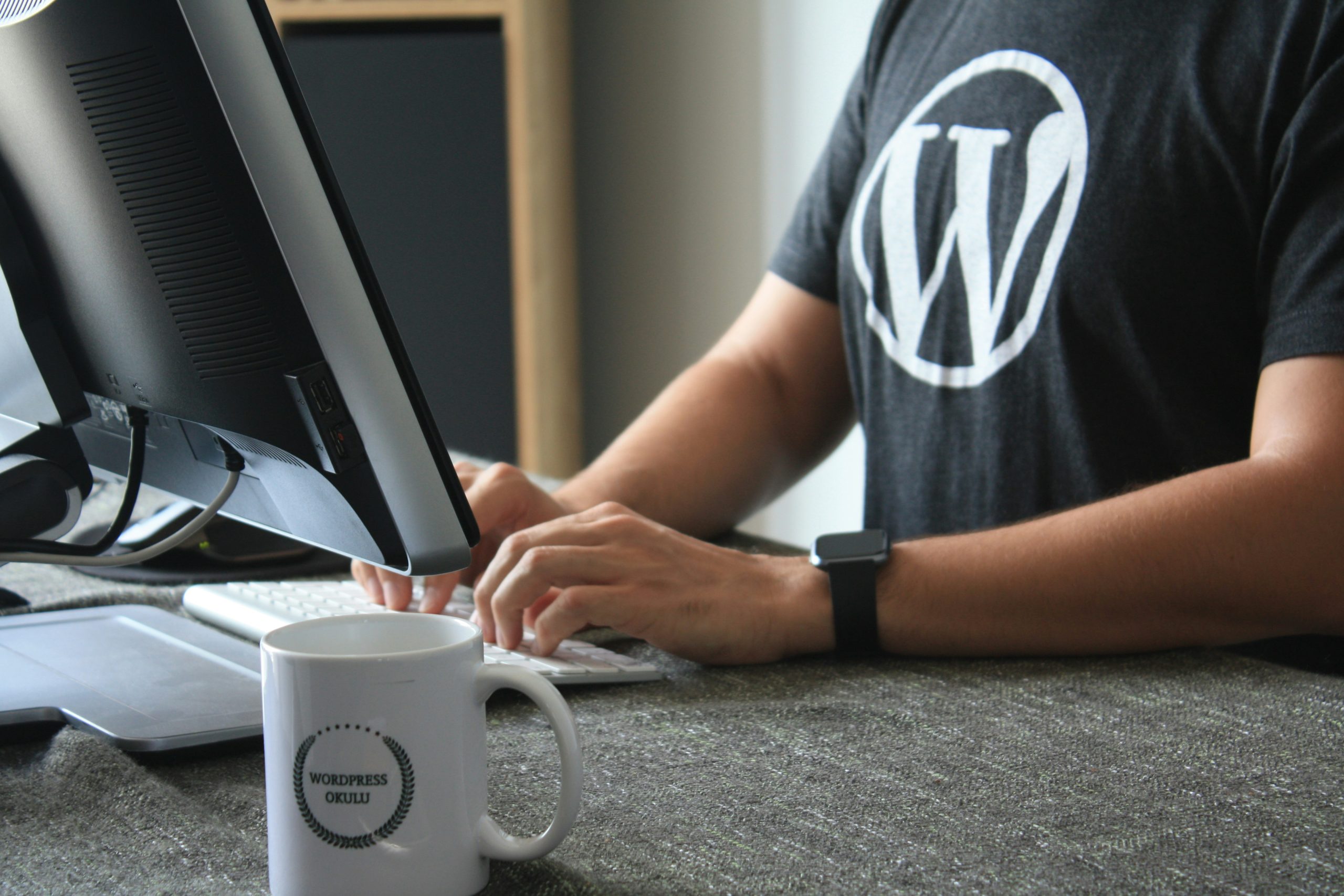
When wp_reset_postdata()
Is Not Needed
While resetting post data is crucial after custom queries, it is unnecessary in certain cases:
- When using the main query: The default WordPress query that loads the main post content does not require resetting.
- When using
get_posts()
: UnlikeWP_Query
,get_posts()
does not modify the global$post
variable, makingwp_reset_postdata()
redundant. - For queries outside the loop: If the data fetched by
WP_Query
is used outside the standard post loop (e.g., in widgets or sidebars), resetting post data is unnecessary unless modifying global post variables.
Alternative: wp_reset_query()
Another function, wp_reset_query()
, serves a different purpose. While wp_reset_postdata()
restores only the global $post
variable, wp_reset_query()
resets the entire query, restoring the original query_posts()
values. Use it when replacing the main query with query_posts()
, but be cautious as query_posts()
itself is generally discouraged in modern WordPress development.
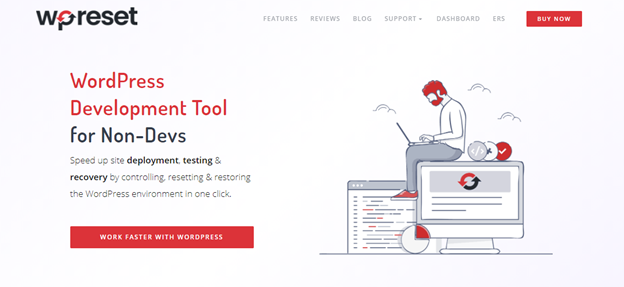
Ensuring Best Practices
To make the best use of wp_reset_postdata()
, adhere to the following best practices:
- Always use
wp_reset_postdata()
after a customWP_Query
. - When using multiple loops on a page, reset post data after each query.
- Avoid modifying the default query unless necessary and always reset if you do.
Conclusion
Using wp_reset_postdata()
correctly is vital to maintaining the integrity of your WordPress loops. Without it, custom queries can interfere with subsequent content, leading to inconsistent or incorrect post displays. Whether you’re dealing with multiple queries on a page or manipulating post data within templates, always ensure that you reset post data to restore WordPress’s global variables to their expected state.